Google Cloud Messaging for Android (GCM) is a free service that helps developers send data from servers to their Android applications on Android devices, and upstream messages from the user's device back to the cloud. This could be a lightweight message telling the Android application that there is new data to be fetched from the server (for instance, a "new email" notification informing the application that it is out of sync with the back end), or it could be a message containing up to 4kb of payload data (so apps like instant messaging can consume the message directly). The GCM service handles all aspects of queueing of messages and delivery to the target Android application running on the target device.
To jump right into using GCM with your Android applications, see Getting Started.
Here are the primary characteristics of Google Cloud Messaging (GCM):
- It allows 3rd-party application servers to send messages to their Android applications.
- Using the GCM Cloud Connection Server, you can receive upstream messages from the user's device.
- An Android application on an Android device doesn't need to be running to receive messages. The system will wake up the Android application via Intent broadcast when the message arrives, as long as the application is set up with the proper broadcast receiver and permissions.
- It does not provide any built-in user interface or other handling for message data. GCM simply passes raw message data received straight to the Android application, which has full control of how to handle it. For example, the application might post a notification, display a custom user interface, or silently sync data.
- It requires devices running Android 2.2 or higher that also have the Google Play Store application installed, or or an emulator running Android 2.2 with Google APIs. However, you are not limited to deploying your Android applications through Google Play Store.
- It uses an existing connection for Google services. For pre-3.0 devices, this requires users to set up their Google account on their mobile devices. A Google account is not a requirement on devices running Android 4.0.4 or higher.
Key Concepts
This table summarizes the key terms and concepts involved in GCM. It is divided into these categories:
- Components — The entities that play a primary role in GCM.
- Credentials — The IDs and tokens that are used in different stages of GCM to ensure that all parties have been authenticated, and that the message is going to the correct place.
Table 1. GCM components and credentials.
Components | |
---|---|
Client App | The GCM-enabled Android application that is running on a device. This must be a 2.2 Android device that has Google Play Store installed, and it must have at least one logged in Google account if the device is running a version lower than Android 4.0.4. Alternatively, for testing you can use an emulator running Android 2.2 with Google APIs. |
3rd-party Application Server | An application server that you write as part of implementing GCM. The 3rd-party application server sends data to an Android application on the device via the GCM connection server. |
GCM Connection Servers | The Google-provided servers involved in taking messages from the 3rd-party application server and sending them to the device. |
Credentials | |
Sender ID | A project number you acquire from the API console, as described in Getting Started. The sender ID is used in the registration process to identify a 3rd-party application server that is permitted to send messages to the device. |
Application ID | The Android application that is registering to receive messages. The Android application is identified by the package name from the manifest. This ensures that the messages are targeted to the correct Android application. |
Registration ID | An ID issued by the GCM servers to the Android application that allows
it to receive messages. Once the Android application has the registration ID, it sends
it to the 3rd-party application server, which uses it to identify each device
that has registered to receive messages for a given Android application. In other words,
a registration ID is tied to a particular Android application running on a particular
device.
Note: If you use backup and restore, you should explicitly avoid backing up registration IDs. When you back up a device, apps back up shared prefs indiscriminately. If you don't explicitly exclude the GCM registration ID, it could get reused on a new device, which would cause delivery errors. |
Google User Account | For GCM to work, the mobile device must include at least one Google account if the device is running a version lower than Android 4.0.4. |
Sender Auth Token | An API key that is saved on the 3rd-party application server that gives the application server authorized access to Google services. The API key is included in the header of POST requests that send messages. |
Architectural Overview
A GCM implementation includes a Google-provided connection server, a 3rd-party app server that interacts with the connection server, and a GCM-enabled client app running on an Android device:
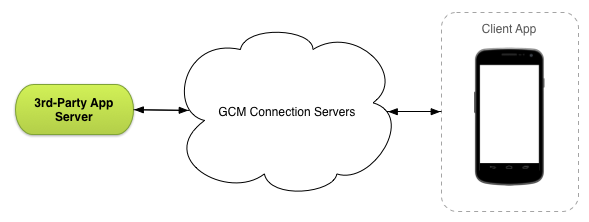
Figure 1. GCM Architecture.
This is how these components interact:
- Google-provided GCM Connection Servers take messages from a 3rd-party application server and send these messages to a GCM-enabled Android application (the "client app") running on a device. Currently Google provides connection servers for HTTP and XMPP.
- The 3rd-Party Application Server is a component that you implement to work with your chosen GCM connection server(s). App servers send messages to a GCM connection server; the connection server enqueues and stores the message, and then sends it to the device when the device is online. For more information, see Implementing GCM Server.
- The Client App is a GCM-enabled Android application running on a device. To receive GCM messages, this app must register with GCM and get a registration ID. If you are using the XMPP (CCS) connection server, the client app can send "upstream" messages back to the connection server. For more information on how to implement the client app, see Implementing GCM Client.
Lifecycle Flow
- Enable GCM. An Android application running on a mobile device registers to receive messages.
- Send a message. A 3rd-party application server sends messages to the device.
- Receive a message. An Android application receives a message from a GCM server.
These processes are described in more detail below.
Enable GCM
The first time the Android application needs to use the messaging service, it
calls the
GoogleCloudMessaging
method register()
, as discussed in
Implementing GCM Client.
The register()
method returns a registration ID. The Android
application should store this ID for later use (for instance,
to check in onCreate()
if it is already registered).
Send a message
Here is the sequence of events that occurs when the application server sends a message:
- The application server sends a message to GCM servers.
- Google enqueues and stores the message in case the device is offline.
- When the device is online, Google sends the message to the device.
- On the device, the system broadcasts the message to the specified Android application via Intent broadcast with proper permissions, so that only the targeted Android application gets the message. This wakes the Android application up. The Android application does not need to be running beforehand to receive the message.
- The Android application processes the message. If the Android application is doing
non-trivial processing, you may want to grab a
PowerManager.WakeLock
and do any processing in a service.
An Android application can unregister GCM if it no longer wants to receive messages.
Receive a message
This is the sequence of events that occurs when an Android application installed on a mobile device receives a message:
- The system receives the incoming message and extracts the raw key/value pairs from the message payload, if any.
- The system passes the key/value pairs to the targeted Android application
in a
com.google.android.c2dm.intent.RECEIVE
Intent as a set of extras. - The Android application extracts the raw data
from the
com.google.android.c2dm.intent.RECEIVE